#import os
from sympy import *
import numpy as np
from tabulate import tabulate
from scipy import signal
import matplotlib.pyplot as plt
import pandas as pd
import SymMNA
from IPython.display import display, Markdown, Math, Latex
init_printing()
22 Thevenin equivalent circuit
22.1 Introduction
The Thevenin equivalent circuit is the reduction of a linear one port circuit to a single source and impedance and is based on Thevenin’s Theorem. This notebook describes solving problem 11.25, given in chapter 11 of Johnson, Hilburn, and Johnson (1978). The Python libraries of SimPy and NumPy are used to perform the math in the proposed solution. The problem asks the student to replace the circuit to the left of terminals a-b by its Thevenin equivalent and find V. The schematic was drawn using LTspice and the nodes were numbered. Terminals a-b are across the resistor R3. The circuit given in the textbook does not include a reference node, however the node at the bottom of the schematic was chosen as the reference node, ground.
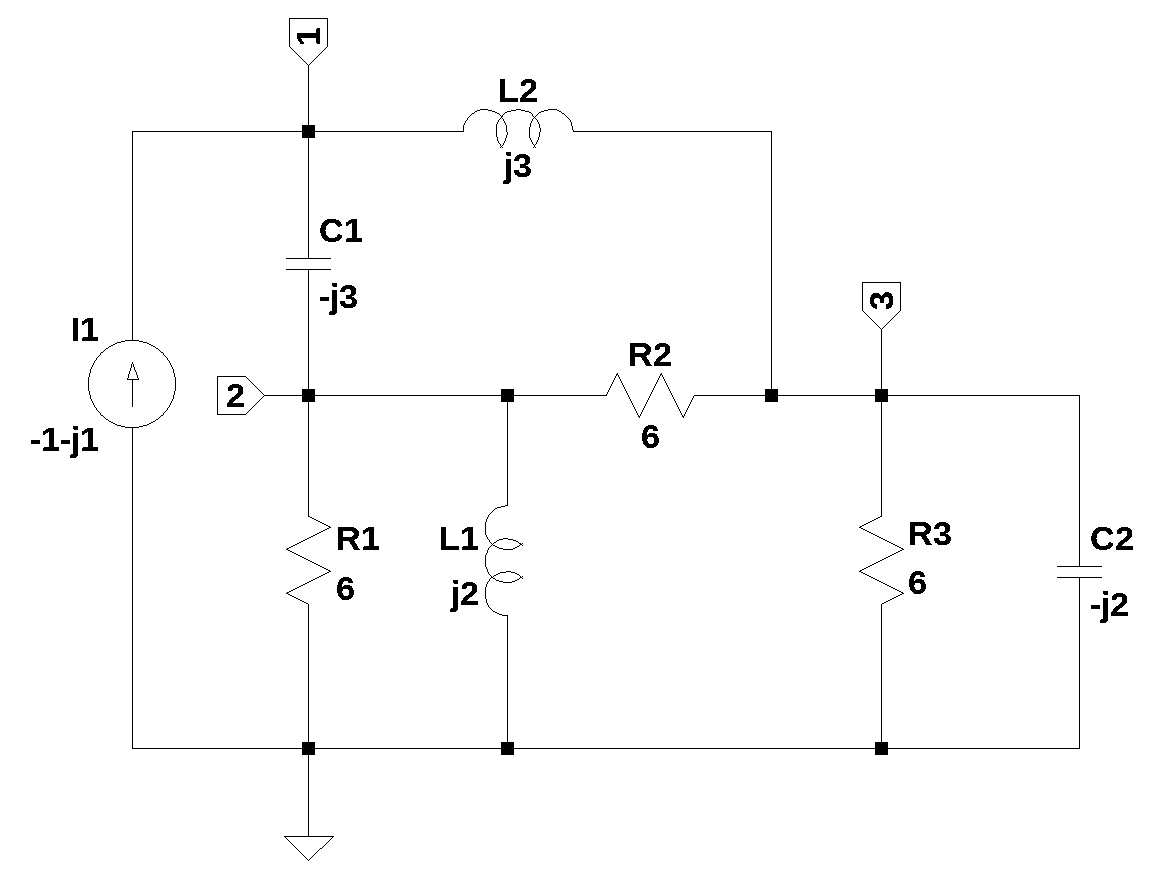
Having drawn the circuit in LTspice, the following netlist was exported as a text file.
R1 2 0 6
R2 3 2 6
R3 0 3 6
L1 2 0 j2
L2 1 3 j3
C1 1 2 -j3
C2 3 0 -j2
I1 0 1 -1-j1
The component values for the inductors and capacitors are complex as well as the value of the current source. It is assumed that the impedance of the inductors and capacitors are at a frequency of 1 radian per second. So accordingly, the values in the net list used by the Python code to generate the network equations has been adjusted as follows:
R1 2 0 6
R2 3 2 6
R3 0 3 6
L1 2 0 2
L2 1 3 3
C1 1 2 0.33
C2 3 0 0.5
I1 0 1 1
The component values are not used when the symbolic network equations are generated, only the reference designators, R1, C1, L1 etc. are used.
22.2 Find the open circuit voltage, Voc
Removing R3 and C2 from the netlist, this gives Voc = V3. The net list is:
I1 0 1 1
R1 2 0 6
R2 3 2 6
*R3 0 3 6
L1 2 0 2
L2 1 3 3
C1 1 2 0.33
*C2 3 0 0.5
Load the net list
= '''
net_list I1 0 1 1
R1 2 0 6
R2 3 2 6
*R3 0 3 6
L1 2 0 2
L2 1 3 3
C1 1 2 0.3333333333
*C2 3 0 0.5
'''
Call the symbolic modified nodal analysis function
= SymMNA.smna(net_list) report, network_df, i_unk_df, A, X, Z
Display the equations
# reform X and Z into Matrix type for printing
= Matrix(X)
Xp = Matrix(Z)
Zp = ''
temp for i in range(len(X)):
+= '${:s}$<br>'.format(latex(Eq((A*Xp)[i:i+1][0],Zp[i])))
temp
Markdown(temp)
\(C_{1} s v_{1} - C_{1} s v_{2} + I_{L2} = I_{1}\)
\(- C_{1} s v_{1} + I_{L1} + v_{2} \left(C_{1} s + \frac{1}{R_{2}} + \frac{1}{R_{1}}\right) - \frac{v_{3}}{R_{2}} = 0\)
\(- I_{L2} - \frac{v_{2}}{R_{2}} + \frac{v_{3}}{R_{2}} = 0\)
\(- I_{L1} L_{1} s + v_{2} = 0\)
\(- I_{L2} L_{2} s + v_{1} - v_{3} = 0\)
Build the network equation matrix
# Put matrices into SymPy
= Matrix(X)
X = Matrix(Z)
Z
= Eq(A*X,Z)
NE_sym NE_sym
\(\displaystyle \left[\begin{matrix}C_{1} s v_{1} - C_{1} s v_{2} + I_{L2}\\- C_{1} s v_{1} + I_{L1} + v_{2} \left(C_{1} s + \frac{1}{R_{2}} + \frac{1}{R_{1}}\right) - \frac{v_{3}}{R_{2}}\\- I_{L2} - \frac{v_{2}}{R_{2}} + \frac{v_{3}}{R_{2}}\\- I_{L1} L_{1} s + v_{2}\\- I_{L2} L_{2} s + v_{1} - v_{3}\end{matrix}\right] = \left[\begin{matrix}I_{1}\\0\\0\\0\\0\end{matrix}\right]\)
# turn the free symbols into SymPy variables
str(NE_sym.free_symbols).replace('{','').replace('}','')) var(
\(\displaystyle \left( L_{2}, \ L_{1}, \ v_{1}, \ R_{1}, \ C_{1}, \ I_{L1}, \ I_{1}, \ s, \ v_{2}, \ I_{L2}, \ v_{3}, \ R_{2}\right)\)
Generate the symbolic solution
= solve(NE_sym,X) U_sym
Display the symbolic solution
= ''
temp for i in U_sym.keys():
+= '${:s} = {:s}$<br>'.format(latex(i),latex(U_sym[i]))
temp
Markdown(temp)
\(v_{1} = \frac{C_{1} I_{1} L_{1} L_{2} R_{1} s^{3} + C_{1} I_{1} L_{1} R_{1} R_{2} s^{2} + I_{1} L_{1} L_{2} s^{2} + I_{1} L_{1} R_{1} s + I_{1} L_{1} R_{2} s + I_{1} L_{2} R_{1} s + I_{1} R_{1} R_{2}}{C_{1} L_{1} L_{2} s^{3} + C_{1} L_{1} R_{2} s^{2} + C_{1} L_{2} R_{1} s^{2} + C_{1} R_{1} R_{2} s + L_{1} s + R_{1}}\)
\(v_{2} = \frac{I_{1} L_{1} R_{1} s}{L_{1} s + R_{1}}\)
\(v_{3} = \frac{C_{1} I_{1} L_{1} L_{2} R_{1} s^{3} + C_{1} I_{1} L_{1} R_{1} R_{2} s^{2} + I_{1} L_{1} R_{1} s + I_{1} L_{1} R_{2} s + I_{1} R_{1} R_{2}}{C_{1} L_{1} L_{2} s^{3} + C_{1} L_{1} R_{2} s^{2} + C_{1} L_{2} R_{1} s^{2} + C_{1} R_{1} R_{2} s + L_{1} s + R_{1}}\)
\(I_{L1} = \frac{I_{1} R_{1}}{L_{1} s + R_{1}}\)
\(I_{L2} = \frac{I_{1}}{C_{1} L_{2} s^{2} + C_{1} R_{2} s + 1}\)
Construct a dictionary of element values
= SymMNA.get_part_values(network_df)
element_values element_values
\(\displaystyle \left\{ C_{1} : 0.3333333333, \ I_{1} : 1.0, \ L_{1} : 2.0, \ L_{2} : 3.0, \ R_{1} : 6.0, \ R_{2} : 6.0\right\}\)
To solve numerically, replace symbols with the element values and the Laplace variable, s, with \(j\omega\) where \(\omega=1\).
#NE = NE_sym.subs({L1:2, L2:3, R1:6, R2:6, C1:1/3, I1:-1-1j, s:1j})
= NE_sym.subs({s:1j,I1:-1-1j})
NE = NE.subs(element_values)
NE NE
\(\displaystyle \left[\begin{matrix}I_{L2} + 0.3333333333 i v_{1} - 0.3333333333 i v_{2}\\I_{L1} - 0.3333333333 i v_{1} + v_{2} \cdot \left(0.333333333333333 + 0.3333333333 i\right) - 0.166666666666667 v_{3}\\- I_{L2} - 0.166666666666667 v_{2} + 0.166666666666667 v_{3}\\- 2.0 i I_{L1} + v_{2}\\- 3.0 i I_{L2} + v_{1} - v_{3}\end{matrix}\right] = \left[\begin{matrix}-1.0 - 1.0 i\\0\\0\\0\\0\end{matrix}\right]\)
Solving the system of equations for the open circuit voltage at node v3.
= solve(NE,X)[v3]
Voc Voc
\(\displaystyle -1.8 + 0.6 i\)
22.3 Find the short circuit current, Isc
Remove C2 and R3, set node 3 to zero, find current in L2 and R2. New net list:
I1 0 1 1
R1 2 0 6
R2 0 2 6
L1 2 0 2
L2 1 0 3
C1 1 2 0.33
= '''
net_list I1 0 1 1
R1 2 0 6
R2 0 2 6
L1 2 0 2
L2 1 0 3
C1 1 2 0.33333333333333
'''
Call the symbolic modified nodal analysis function
= SymMNA.smna(net_list) report, network_df, i_unk_df, A, X, Z
Display the equations
# reform X and Z into Matrix type for printing
= Matrix(X)
Xp = Matrix(Z)
Zp = ''
temp for i in range(len(X)):
+= '${:s}$<br>'.format(latex(Eq((A*Xp)[i:i+1][0],Zp[i])))
temp
Markdown(temp)
\(C_{1} s v_{1} - C_{1} s v_{2} + I_{L2} = I_{1}\)
\(- C_{1} s v_{1} + I_{L1} + v_{2} \left(C_{1} s + \frac{1}{R_{2}} + \frac{1}{R_{1}}\right) = 0\)
\(- I_{L1} L_{1} s + v_{2} = 0\)
\(- I_{L2} L_{2} s + v_{1} = 0\)
Build the network equation matrix
# Put matrices into SymPy
= Matrix(X)
X = Matrix(Z)
Z
= Eq(A*X,Z)
NE_sym NE_sym
\(\displaystyle \left[\begin{matrix}C_{1} s v_{1} - C_{1} s v_{2} + I_{L2}\\- C_{1} s v_{1} + I_{L1} + v_{2} \left(C_{1} s + \frac{1}{R_{2}} + \frac{1}{R_{1}}\right)\\- I_{L1} L_{1} s + v_{2}\\- I_{L2} L_{2} s + v_{1}\end{matrix}\right] = \left[\begin{matrix}I_{1}\\0\\0\\0\end{matrix}\right]\)
# turn the free symbols into SymPy variables
str(NE_sym.free_symbols).replace('{','').replace('}','')) var(
\(\displaystyle \left( L_{2}, \ L_{1}, \ v_{1}, \ R_{1}, \ C_{1}, \ I_{L1}, \ I_{1}, \ s, \ v_{2}, \ I_{L2}, \ R_{2}\right)\)
Symbolic solution
= solve(NE_sym,X) U_sym
Display the symbolic solution
= ''
temp for i in U_sym.keys():
+= '${:s} = {:s}$<br>'.format(latex(i),latex(U_sym[i]))
temp
Markdown(temp)
\(v_{1} = \frac{C_{1} I_{1} L_{1} L_{2} R_{1} R_{2} s^{3} + I_{1} L_{1} L_{2} R_{1} s^{2} + I_{1} L_{1} L_{2} R_{2} s^{2} + I_{1} L_{2} R_{1} R_{2} s}{C_{1} L_{1} L_{2} R_{1} s^{3} + C_{1} L_{1} L_{2} R_{2} s^{3} + C_{1} L_{1} R_{1} R_{2} s^{2} + C_{1} L_{2} R_{1} R_{2} s^{2} + L_{1} R_{1} s + L_{1} R_{2} s + R_{1} R_{2}}\)
\(v_{2} = \frac{C_{1} I_{1} L_{1} L_{2} R_{1} R_{2} s^{3}}{C_{1} L_{1} L_{2} R_{1} s^{3} + C_{1} L_{1} L_{2} R_{2} s^{3} + C_{1} L_{1} R_{1} R_{2} s^{2} + C_{1} L_{2} R_{1} R_{2} s^{2} + L_{1} R_{1} s + L_{1} R_{2} s + R_{1} R_{2}}\)
\(I_{L1} = \frac{C_{1} I_{1} L_{2} R_{1} R_{2} s^{2}}{C_{1} L_{1} L_{2} R_{1} s^{3} + C_{1} L_{1} L_{2} R_{2} s^{3} + C_{1} L_{1} R_{1} R_{2} s^{2} + C_{1} L_{2} R_{1} R_{2} s^{2} + L_{1} R_{1} s + L_{1} R_{2} s + R_{1} R_{2}}\)
\(I_{L2} = \frac{C_{1} I_{1} L_{1} R_{1} R_{2} s^{2} + I_{1} L_{1} R_{1} s + I_{1} L_{1} R_{2} s + I_{1} R_{1} R_{2}}{C_{1} L_{1} L_{2} R_{1} s^{3} + C_{1} L_{1} L_{2} R_{2} s^{3} + C_{1} L_{1} R_{1} R_{2} s^{2} + C_{1} L_{2} R_{1} R_{2} s^{2} + L_{1} R_{1} s + L_{1} R_{2} s + R_{1} R_{2}}\)
/R2 + U_sym[I_L2] U_sym[v2]
\(\displaystyle \frac{C_{1} I_{1} L_{1} L_{2} R_{1} s^{3}}{C_{1} L_{1} L_{2} R_{1} s^{3} + C_{1} L_{1} L_{2} R_{2} s^{3} + C_{1} L_{1} R_{1} R_{2} s^{2} + C_{1} L_{2} R_{1} R_{2} s^{2} + L_{1} R_{1} s + L_{1} R_{2} s + R_{1} R_{2}} + \frac{C_{1} I_{1} L_{1} R_{1} R_{2} s^{2} + I_{1} L_{1} R_{1} s + I_{1} L_{1} R_{2} s + I_{1} R_{1} R_{2}}{C_{1} L_{1} L_{2} R_{1} s^{3} + C_{1} L_{1} L_{2} R_{2} s^{3} + C_{1} L_{1} R_{1} R_{2} s^{2} + C_{1} L_{2} R_{1} R_{2} s^{2} + L_{1} R_{1} s + L_{1} R_{2} s + R_{1} R_{2}}\)
Construct a dictionary of element values
= SymMNA.get_part_values(network_df)
element_values element_values
\(\displaystyle \left\{ C_{1} : 0.33333333333333, \ I_{1} : 1.0, \ L_{1} : 2.0, \ L_{2} : 3.0, \ R_{1} : 6.0, \ R_{2} : 6.0\right\}\)
#NE = NE_sym.subs({L1:2, L2:3, R1:6, R2:6, C1:1/3, I1:-1-1j, s:1j})
= NE_sym.subs({s:1j,I1:-1-1j})
NE = NE.subs(element_values)
NE NE
\(\displaystyle \left[\begin{matrix}I_{L2} + 0.33333333333333 i v_{1} - 0.33333333333333 i v_{2}\\I_{L1} - 0.33333333333333 i v_{1} + v_{2} \cdot \left(0.333333333333333 + 0.33333333333333 i\right)\\- 2.0 i I_{L1} + v_{2}\\- 3.0 i I_{L2} + v_{1}\end{matrix}\right] = \left[\begin{matrix}-1.0 - 1.0 i\\0\\0\\0\end{matrix}\right]\)
= solve(NE,X)
U U
\(\displaystyle \left\{ I_{L1} : -1.5 - 1.5 i, \ I_{L2} : -0.5 + 1.5 i, \ v_{1} : -4.5 - 1.5 i, \ v_{2} : 3.0 - 3.0 i\right\}\)
= U[v2]/6 + U[I_L2]
Isc Isc
\(\displaystyle 1.0 i\)
= simplify(Voc/Isc)
Zth Zth
\(\displaystyle 0.6 + 1.8 i\)
Current in I_R2 = V2/R2
= U[v2]/6 + U[I_L2]
Isc Isc
\(\displaystyle 1.0 i\)
= simplify(Voc/Isc)
Zth Zth
\(\displaystyle 0.6 + 1.8 i\)
Voc along with Zth are the values to the Thevenin equivalent circuit.
22.4 Find V3 using the Thevenin equivalent circuit
The load attached to the Thevenin equivalent circuit is the parallel of the resistor and capacitor.
\(Z = \frac{1}{\frac{1}{R}+\frac{1}{C}}\)
The load \(Z\) is the parallel combination of R = 6 and C = -j2.
= (1)/(1/(6)+1/(-2j))
Z Z
(0.6-1.7999999999999998j)
Using the values for \(V_{oc}\) and \(Z_{th}\) obtained above, we write the equation for V3 as a voltage divider and have SymPy simplify the result.
*Voc/(Zth+Z)) simplify(Z
\(\displaystyle 4.62592926927148 \cdot 10^{-16} + 3.0 i\)
We can get SymPy to ignore small numbers by using the round function set to 3 digits.
*Voc/(Zth+Z)).round(3) simplify(Z
\(\displaystyle 3.0 i\)
22.5 Find V3 using the complete circuit
Checking the answer for V3, by solving the equations for the complete circuit. The net list is:
I1 0 1 1
R1 2 0 6
R2 3 2 6
R3 0 3 6
L1 2 0 2
L2 1 3 3
C1 1 2 0.33
C2 3 0 0.5
Declare the symbols and equations for the complete circuit.
= '''
net_list I1 0 1 1
R1 2 0 6
R2 3 2 6
R3 0 3 6
L1 2 0 2
L2 1 3 3
C1 1 2 0.333333333333333
C2 3 0 0.5
'''
Call the symbolic modified nodal analysis function
= SymMNA.smna(net_list) report, network_df, i_unk_df, A, X, Z
Display the equations
# reform X and Z into Matrix type for printing
= Matrix(X)
Xp = Matrix(Z)
Zp = ''
temp for i in range(len(X)):
+= '${:s}$<br>'.format(latex(Eq((A*Xp)[i:i+1][0],Zp[i])))
temp
Markdown(temp)
\(C_{1} s v_{1} - C_{1} s v_{2} + I_{L2} = I_{1}\)
\(- C_{1} s v_{1} + I_{L1} + v_{2} \left(C_{1} s + \frac{1}{R_{2}} + \frac{1}{R_{1}}\right) - \frac{v_{3}}{R_{2}} = 0\)
\(- I_{L2} + v_{3} \left(C_{2} s + \frac{1}{R_{3}} + \frac{1}{R_{2}}\right) - \frac{v_{2}}{R_{2}} = 0\)
\(- I_{L1} L_{1} s + v_{2} = 0\)
\(- I_{L2} L_{2} s + v_{1} - v_{3} = 0\)
Build the network equation matrix
# Put matrices into SymPy
= Matrix(X)
X = Matrix(Z)
Z
= Eq(A*X,Z)
NE_sym NE_sym
\(\displaystyle \left[\begin{matrix}C_{1} s v_{1} - C_{1} s v_{2} + I_{L2}\\- C_{1} s v_{1} + I_{L1} + v_{2} \left(C_{1} s + \frac{1}{R_{2}} + \frac{1}{R_{1}}\right) - \frac{v_{3}}{R_{2}}\\- I_{L2} + v_{3} \left(C_{2} s + \frac{1}{R_{3}} + \frac{1}{R_{2}}\right) - \frac{v_{2}}{R_{2}}\\- I_{L1} L_{1} s + v_{2}\\- I_{L2} L_{2} s + v_{1} - v_{3}\end{matrix}\right] = \left[\begin{matrix}I_{1}\\0\\0\\0\\0\end{matrix}\right]\)
# turn the free symbols into SymPy variables
str(NE_sym.free_symbols).replace('{','').replace('}','')) var(
\(\displaystyle \left( L_{2}, \ L_{1}, \ v_{1}, \ R_{1}, \ C_{1}, \ I_{L1}, \ I_{1}, \ R_{3}, \ s, \ v_{2}, \ I_{L2}, \ v_{3}, \ R_{2}, \ C_{2}\right)\)
Symbolic solution
= solve(NE_sym,X) U_sym
Display the symbolic solution
= ''
temp for i in U_sym.keys():
+= '${:s} = {:s}$<br>'.format(latex(i),latex(U_sym[i]))
temp
Markdown(temp)
\(v_{1} = \frac{C_{1} C_{2} I_{1} L_{1} L_{2} R_{1} R_{2} R_{3} s^{4} + C_{1} I_{1} L_{1} L_{2} R_{1} R_{2} s^{3} + C_{1} I_{1} L_{1} L_{2} R_{1} R_{3} s^{3} + C_{1} I_{1} L_{1} R_{1} R_{2} R_{3} s^{2} + C_{2} I_{1} L_{1} L_{2} R_{1} R_{3} s^{3} + C_{2} I_{1} L_{1} L_{2} R_{2} R_{3} s^{3} + C_{2} I_{1} L_{2} R_{1} R_{2} R_{3} s^{2} + I_{1} L_{1} L_{2} R_{1} s^{2} + I_{1} L_{1} L_{2} R_{2} s^{2} + I_{1} L_{1} L_{2} R_{3} s^{2} + I_{1} L_{1} R_{1} R_{3} s + I_{1} L_{1} R_{2} R_{3} s + I_{1} L_{2} R_{1} R_{2} s + I_{1} L_{2} R_{1} R_{3} s + I_{1} R_{1} R_{2} R_{3}}{C_{1} C_{2} L_{1} L_{2} R_{1} R_{3} s^{4} + C_{1} C_{2} L_{1} L_{2} R_{2} R_{3} s^{4} + C_{1} C_{2} L_{1} R_{1} R_{2} R_{3} s^{3} + C_{1} C_{2} L_{2} R_{1} R_{2} R_{3} s^{3} + C_{1} L_{1} L_{2} R_{1} s^{3} + C_{1} L_{1} L_{2} R_{2} s^{3} + C_{1} L_{1} L_{2} R_{3} s^{3} + C_{1} L_{1} R_{1} R_{2} s^{2} + C_{1} L_{1} R_{2} R_{3} s^{2} + C_{1} L_{2} R_{1} R_{2} s^{2} + C_{1} L_{2} R_{1} R_{3} s^{2} + C_{1} R_{1} R_{2} R_{3} s + C_{2} L_{1} R_{1} R_{3} s^{2} + C_{2} L_{1} R_{2} R_{3} s^{2} + C_{2} R_{1} R_{2} R_{3} s + L_{1} R_{1} s + L_{1} R_{2} s + L_{1} R_{3} s + R_{1} R_{2} + R_{1} R_{3}}\)
\(v_{2} = \frac{C_{1} C_{2} I_{1} L_{1} L_{2} R_{1} R_{2} R_{3} s^{4} + C_{1} I_{1} L_{1} L_{2} R_{1} R_{2} s^{3} + C_{1} I_{1} L_{1} L_{2} R_{1} R_{3} s^{3} + C_{1} I_{1} L_{1} R_{1} R_{2} R_{3} s^{2} + I_{1} L_{1} R_{1} R_{3} s}{C_{1} C_{2} L_{1} L_{2} R_{1} R_{3} s^{4} + C_{1} C_{2} L_{1} L_{2} R_{2} R_{3} s^{4} + C_{1} C_{2} L_{1} R_{1} R_{2} R_{3} s^{3} + C_{1} C_{2} L_{2} R_{1} R_{2} R_{3} s^{3} + C_{1} L_{1} L_{2} R_{1} s^{3} + C_{1} L_{1} L_{2} R_{2} s^{3} + C_{1} L_{1} L_{2} R_{3} s^{3} + C_{1} L_{1} R_{1} R_{2} s^{2} + C_{1} L_{1} R_{2} R_{3} s^{2} + C_{1} L_{2} R_{1} R_{2} s^{2} + C_{1} L_{2} R_{1} R_{3} s^{2} + C_{1} R_{1} R_{2} R_{3} s + C_{2} L_{1} R_{1} R_{3} s^{2} + C_{2} L_{1} R_{2} R_{3} s^{2} + C_{2} R_{1} R_{2} R_{3} s + L_{1} R_{1} s + L_{1} R_{2} s + L_{1} R_{3} s + R_{1} R_{2} + R_{1} R_{3}}\)
\(v_{3} = \frac{C_{1} I_{1} L_{1} L_{2} R_{1} R_{3} s^{3} + C_{1} I_{1} L_{1} R_{1} R_{2} R_{3} s^{2} + I_{1} L_{1} R_{1} R_{3} s + I_{1} L_{1} R_{2} R_{3} s + I_{1} R_{1} R_{2} R_{3}}{C_{1} C_{2} L_{1} L_{2} R_{1} R_{3} s^{4} + C_{1} C_{2} L_{1} L_{2} R_{2} R_{3} s^{4} + C_{1} C_{2} L_{1} R_{1} R_{2} R_{3} s^{3} + C_{1} C_{2} L_{2} R_{1} R_{2} R_{3} s^{3} + C_{1} L_{1} L_{2} R_{1} s^{3} + C_{1} L_{1} L_{2} R_{2} s^{3} + C_{1} L_{1} L_{2} R_{3} s^{3} + C_{1} L_{1} R_{1} R_{2} s^{2} + C_{1} L_{1} R_{2} R_{3} s^{2} + C_{1} L_{2} R_{1} R_{2} s^{2} + C_{1} L_{2} R_{1} R_{3} s^{2} + C_{1} R_{1} R_{2} R_{3} s + C_{2} L_{1} R_{1} R_{3} s^{2} + C_{2} L_{1} R_{2} R_{3} s^{2} + C_{2} R_{1} R_{2} R_{3} s + L_{1} R_{1} s + L_{1} R_{2} s + L_{1} R_{3} s + R_{1} R_{2} + R_{1} R_{3}}\)
\(I_{L1} = \frac{C_{1} C_{2} I_{1} L_{2} R_{1} R_{2} R_{3} s^{3} + C_{1} I_{1} L_{2} R_{1} R_{2} s^{2} + C_{1} I_{1} L_{2} R_{1} R_{3} s^{2} + C_{1} I_{1} R_{1} R_{2} R_{3} s + I_{1} R_{1} R_{3}}{C_{1} C_{2} L_{1} L_{2} R_{1} R_{3} s^{4} + C_{1} C_{2} L_{1} L_{2} R_{2} R_{3} s^{4} + C_{1} C_{2} L_{1} R_{1} R_{2} R_{3} s^{3} + C_{1} C_{2} L_{2} R_{1} R_{2} R_{3} s^{3} + C_{1} L_{1} L_{2} R_{1} s^{3} + C_{1} L_{1} L_{2} R_{2} s^{3} + C_{1} L_{1} L_{2} R_{3} s^{3} + C_{1} L_{1} R_{1} R_{2} s^{2} + C_{1} L_{1} R_{2} R_{3} s^{2} + C_{1} L_{2} R_{1} R_{2} s^{2} + C_{1} L_{2} R_{1} R_{3} s^{2} + C_{1} R_{1} R_{2} R_{3} s + C_{2} L_{1} R_{1} R_{3} s^{2} + C_{2} L_{1} R_{2} R_{3} s^{2} + C_{2} R_{1} R_{2} R_{3} s + L_{1} R_{1} s + L_{1} R_{2} s + L_{1} R_{3} s + R_{1} R_{2} + R_{1} R_{3}}\)
\(I_{L2} = \frac{C_{1} C_{2} I_{1} L_{1} R_{1} R_{2} R_{3} s^{3} + C_{1} I_{1} L_{1} R_{1} R_{2} s^{2} + C_{2} I_{1} L_{1} R_{1} R_{3} s^{2} + C_{2} I_{1} L_{1} R_{2} R_{3} s^{2} + C_{2} I_{1} R_{1} R_{2} R_{3} s + I_{1} L_{1} R_{1} s + I_{1} L_{1} R_{2} s + I_{1} L_{1} R_{3} s + I_{1} R_{1} R_{2} + I_{1} R_{1} R_{3}}{C_{1} C_{2} L_{1} L_{2} R_{1} R_{3} s^{4} + C_{1} C_{2} L_{1} L_{2} R_{2} R_{3} s^{4} + C_{1} C_{2} L_{1} R_{1} R_{2} R_{3} s^{3} + C_{1} C_{2} L_{2} R_{1} R_{2} R_{3} s^{3} + C_{1} L_{1} L_{2} R_{1} s^{3} + C_{1} L_{1} L_{2} R_{2} s^{3} + C_{1} L_{1} L_{2} R_{3} s^{3} + C_{1} L_{1} R_{1} R_{2} s^{2} + C_{1} L_{1} R_{2} R_{3} s^{2} + C_{1} L_{2} R_{1} R_{2} s^{2} + C_{1} L_{2} R_{1} R_{3} s^{2} + C_{1} R_{1} R_{2} R_{3} s + C_{2} L_{1} R_{1} R_{3} s^{2} + C_{2} L_{1} R_{2} R_{3} s^{2} + C_{2} R_{1} R_{2} R_{3} s + L_{1} R_{1} s + L_{1} R_{2} s + L_{1} R_{3} s + R_{1} R_{2} + R_{1} R_{3}}\)
U_sym[v3]
\(\displaystyle \frac{C_{1} I_{1} L_{1} L_{2} R_{1} R_{3} s^{3} + C_{1} I_{1} L_{1} R_{1} R_{2} R_{3} s^{2} + I_{1} L_{1} R_{1} R_{3} s + I_{1} L_{1} R_{2} R_{3} s + I_{1} R_{1} R_{2} R_{3}}{C_{1} C_{2} L_{1} L_{2} R_{1} R_{3} s^{4} + C_{1} C_{2} L_{1} L_{2} R_{2} R_{3} s^{4} + C_{1} C_{2} L_{1} R_{1} R_{2} R_{3} s^{3} + C_{1} C_{2} L_{2} R_{1} R_{2} R_{3} s^{3} + C_{1} L_{1} L_{2} R_{1} s^{3} + C_{1} L_{1} L_{2} R_{2} s^{3} + C_{1} L_{1} L_{2} R_{3} s^{3} + C_{1} L_{1} R_{1} R_{2} s^{2} + C_{1} L_{1} R_{2} R_{3} s^{2} + C_{1} L_{2} R_{1} R_{2} s^{2} + C_{1} L_{2} R_{1} R_{3} s^{2} + C_{1} R_{1} R_{2} R_{3} s + C_{2} L_{1} R_{1} R_{3} s^{2} + C_{2} L_{1} R_{2} R_{3} s^{2} + C_{2} R_{1} R_{2} R_{3} s + L_{1} R_{1} s + L_{1} R_{2} s + L_{1} R_{3} s + R_{1} R_{2} + R_{1} R_{3}}\)
Construct a dictionary of element values
element_values
= SymMNA.get_part_values(network_df)
element_values element_values
\(\displaystyle \left\{ C_{1} : 0.333333333333333, \ C_{2} : 0.5, \ I_{1} : 1.0, \ L_{1} : 2.0, \ L_{2} : 3.0, \ R_{1} : 6.0, \ R_{2} : 6.0, \ R_{3} : 6.0\right\}\)
#NE = NE_sym.subs({L1:2, L2:3, R1:6, R2:6, C1:1/3, I1:-1-1j, s:1j})
= NE_sym.subs({s:1j,I1:-1-1j})
NE = NE.subs(element_values)
NE NE
\(\displaystyle \left[\begin{matrix}I_{L2} + 0.333333333333333 i v_{1} - 0.333333333333333 i v_{2}\\I_{L1} - 0.333333333333333 i v_{1} + v_{2} \cdot \left(0.333333333333333 + 0.333333333333333 i\right) - 0.166666666666667 v_{3}\\- I_{L2} - 0.166666666666667 v_{2} + v_{3} \cdot \left(0.333333333333333 + 0.5 i\right)\\- 2.0 i I_{L1} + v_{2}\\- 3.0 i I_{L2} + v_{1} - v_{3}\end{matrix}\right] = \left[\begin{matrix}-1.0 - 1.0 i\\0\\0\\0\\0\end{matrix}\right]\)
Solving the system of equations for v3 using the SymPy solve function.
= solve(NE,X)
U U[v3]
\(\displaystyle 3.0 i\)
The value obtained by solving the system equations for the complete network agrees with the Thevenin equivalent circuit solution.